[java] 대칭 키(AES) 알고리즘을 사용해서 데이터를 Base64 방식으로 인코딩/디코딩하는 방법.
2022. 11. 16. 15:53ㆍLanguage/java
728x90
728x90
-Base64, Encoding, Decoding 설명 참고.
-AES algorithm 설명 참고.
- https://www.crocus.co.kr/1230
- https://veneas.tistory.com/entry/JAVA-%EC%9E%90%EB%B0%94-AES-%EC%95%94%ED%98%B8%ED%99%94-%ED%95%98%EA%B8%B0-AES-128-AES-192-AES-256
-AES key를 사용해서 데이터를 Base64 방식으로 암호화/복호화하는 *.java 코드.
//AES algorithm
import javax.crypto.Cipher;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
//Base64 enc, dec
import org.apache.commons.codec.binary.Base64;
public class aesEncDec {
public static void main(String[] args) {
String Key = "12345678901234567890123456789012";
String encMsg = "abcdeabcdeabcdeabcdeabcdeabcdeabcdeabcdeabcdeabcde";
System.out.println("====================================================");
System.out.println(" Before encMsg () : " + new String(encMsg));
System.out.println("====================================================");
//Encrpytion
try {
encMsg = encrypt(Key, encMsg);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("====================================================");
System.out.println(" After encMsg () : " + new String(encMsg));
System.out.println("====================================================\n");
String decMsg = encMsg;
System.out.println("====================================================");
System.out.println(" Before decMsg () : " + new String(decMsg));
System.out.println("====================================================");
//Decryption
try {
decMsg = decrypt(Key, decMsg);
} catch (Exception e) {
e.printStackTrace();
}
System.out.println("====================================================");
System.out.println(" After decMsg () : " + new String(decMsg));
System.out.println("====================================================");
}
public static String encrypt(String secretKey, String data) throws Exception {
//SecretKey 생성.
SecretKey skey = new SecretKeySpec(secretKey.getBytes(), "AES");
SecretKeySpec skeySpec = new SecretKeySpec(skey.getEncoded(), "AES");
//Cipher 객체 인스턴스화
Cipher cipher = Cipher.getInstance("AES");
//Cipher 객체 초기화
cipher.init(Cipher.ENCRYPT_MODE, skeySpec);
//Encrpytion
byte[] encrypted = cipher.doFinal(data.getBytes("UTF-8"));
return new String(Base64.encodeBase64(encrypted));
}
public static String decrypt(String secretKey, String encrypted)
throws Exception {
//SecretKey 생성.
SecretKey skey = new SecretKeySpec(secretKey.getBytes(), "AES");
SecretKeySpec skeySpec = new SecretKeySpec(skey.getEncoded(), "AES");
//Cipher 객체 인스턴스화
Cipher cipher = Cipher.getInstance("AES");
//Cipher 객체 초기화
cipher.init(Cipher.DECRYPT_MODE, skeySpec);
//Decryption
byte[] encryptedBytes = Base64.decodeBase64(encrypted.getBytes());
byte[] originalBytes = cipher.doFinal(encryptedBytes);
return new String(originalBytes, "UTF-8");
}
}
-Apache Commons lib(org.apache.commons.codec.binary.Base64)을 사용하여, Base64 방식으로 인코딩/디코딩 수행하는 예제이기 때문에, 하단의 링크에서 라이브러리(commons-codec-1.7.jar) 다운로드.
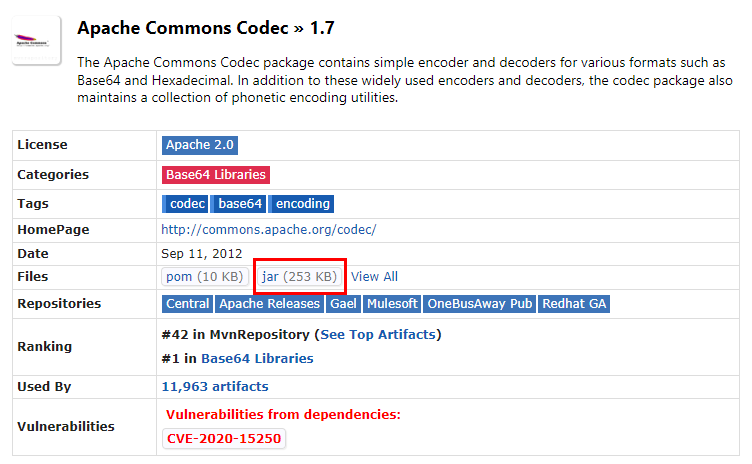
-컴파일 수행.
$ javac -classpath commons-codec-1.7.jar -encoding UTF8 aesEncDec.java
-컴파일한 클래스 실행.
$ java -classpath .;commons-codec-1.7.jar aesEncDec
====================================================
Before encMsg () : abcdeabcdeabcdeabcdeabcdeabcdeabcdeabcdeabcdeabcde
====================================================
====================================================
After encMsg () : +LE3YUHMeJXCOtREOUfuNvZ5udNeLqWzI2elolcx+xO8R5NACufXdK8f8tSlWCA5RNAkI+pVdawUqMs4wt6LwQ==
====================================================
====================================================
Before decMsg () : +LE3YUHMeJXCOtREOUfuNvZ5udNeLqWzI2elolcx+xO8R5NACufXdK8f8tSlWCA5RNAkI+pVdawUqMs4wt6LwQ==
====================================================
====================================================
After decMsg () : abcdeabcdeabcdeabcdeabcdeabcdeabcdeabcdeabcdeabcde
====================================================
728x90
728x90
'Language > java' 카테고리의 다른 글
[Java] JSON의 Key를 파싱하고 Value를 파일로 저장하는 방법. (0) | 2024.11.02 |
---|---|
[Java] jar 파일에 포함된 외부 라이브러리를 제거하는 방법. (0) | 2024.09.27 |
[java] JSch 라이브러리 & DH 알고리즘 사용하여, java sftp client 환경 구축하는 방법.(*.java, sftp client code) (0) | 2022.03.25 |
[java] HTTP POST body data를 송/수신하는 서블릿 코드.(*.java, servlet code) (0) | 2022.02.14 |
[java] TCP/IP socket code(*.java, TCPIP 소켓 코드) (0) | 2022.01.25 |